Hello world 👋🏻 my name is Francisco, fcoteroba on the Internet and today I bring you again a post in which we are going to carry out another project in our dear and beloved 💚 Python programming language 🐍.
This project is somewhat more complex than the previous two already carried out on the web.
If you haven't seen them already, I recommend watching them before continuing with this one: How can we do a calculator in the terminal? and the project where we do a script for generate QR codes.
Before starting, although later I will explain what it is, I will I recommend visiting a post that I uploaded more than a month ago, in which I explain many of the most used computer terms in our day to day. Since, in this post, you will see words that you probably do not sound a lot. 🤯 You can reed the post here.
I also want to remind you that a few months ago I uploaded a video to my YouTube channel, very interesting, focused on home automation. Specifically, we connect, configure and install a smart light bulb 💡 with which you can change its color, turn it off, turn it on and much more simply by using your mobile phone and/or voice assistants such as Google, Alexa, etc. 👇🏻
Now yes, let's start!
What is an exchange?
Well, I imagine that before programming something, you'll want to know what that thing is. To do this, I'll tell you what Wikipedia says about it:
Exchanges, also known as exchange platforms or markets, are digital platforms that allow digital currencies to be exchanged for fiat money and/or other cryptocurrencies or goods.
It is a rare explanation as well as explicit, solely and exclusively focused on cryptocurrencies.
But, in reality, an exchange is defined as a site, website or (in our case) program to enter a value in a certain currency or cryptocurrency.
And that is what we are going to do today, we are going to create a graphical interface, or GUI for its acronym in English, in which the user will insert a value in a certain currency and the program will display its current value in real time.
BEFORE START YOU NEEDS TO KNOW:
An API (a word you'll see in a moment) is (as defined on the Red Hat website) a set of definitions and protocols used to develop and integrate application software. API stands for application programming interface.
In short, an API is usually an independent program file, programmed solely and exclusively to be integrated into other applications.
Hey, how are we going to show the current value, if this is a market that varies every second?
I am going to proceed to explain everything to you, step by step, just as we always do here. But first, I'm going to show you a small GIF of what you are going to learn to program by reading this simple post! 😁

Now yes, here comes the first step 1️⃣.
We must register at ExchanGerate-API, which is a free, open and free API to make conversions between the most diverse currencies.
Registration is simple, we will simply have to write the email to which we want the free key to arrive and click the button.
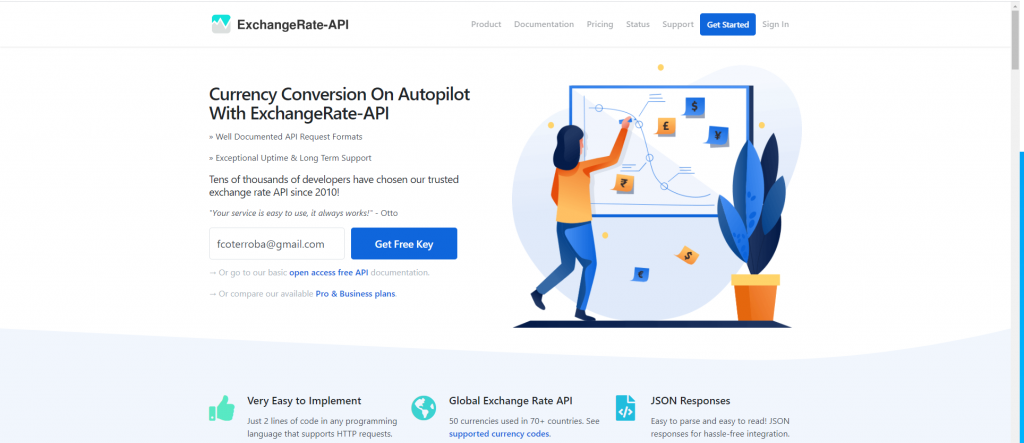
Once we register, a very long number will appear on our dashboard. This number is the API key that we will later use in Python 🐍.
When we have said code. Let's start by opening our favorite IDE. Which in my case is VSCode and we will start importing the packages that we are going to need in this project. 📦
Although I say it every time I make a post about Python, I remember that in this programming language it is not necessary to specify what type the variable will be (Char, float, integer,...), unlike many other programming languages since, Python 🐍, it will automatically understand the type of variable depending on what we save in it.
For this project we are going to import tkinter in the following ways from tkinter import * and from tkinter import ttk. These two will obviously be to create the graphical interface of the program.
Finally, we must also import the requests package, which works with web pages to decode their JSON, extract information and much more. The code is import requests.
IMPORTANT,, you will probably get an error with the requests package, and this is because you have to install it first on the command line using the pip installer. pip install requests
Remember 🧠
Comments in Python are written by putting the symbol at the beginning of the line #
The functions in programming. Functions, in short, are a piece of code that, even if written, will never be activated until it is invoked. 🧙🏻♂️
TKinter 🎨
We have to create a root window by setting a name before Tk().
Later we give the size of the window with minsize(valorx, valory).
We set a title for the window with title(“Nombre”)
And so that no user can enlarge or reduce the size of the window, we will set the two parameters to 0. resizable(x,y).
Adding all this, our program starts like this:
from tkinter import * from tkinter import ttk import requests #Definición ventana ventana = Tk() ventana.minsize(500, 500) ventana.title("Exchange realizado en Python | fcoterroba.com") ventana.resizable(0,0)
We are going to do everything now, everything that will appear in our main window.
1️⃣ Firstly, we are going to write a Label as the title of the program, giving it its respective design and distribution configuration.
titulo_label = Label(ventana, text="¡Exchange de todas las monedas!") titulo_label.config( fg="white", bg="black", font=("Arial", 30), padx=210, pady=20 ) titulo_label.grid(row=0, column=0)
2️⃣ The second thing, establish the text entry, even if it is a number, Tkinter makes no distinction, so we will be the ones who will give an alert if it is not a number.
The text input will be stored in a variable that we must declare previously. I have done it right on the line below ventana.resizable
numero_label = Label(ventana, text="Dime el número y la moneda correspondiente") numero_entry = Entry(ventana, textvariable=numero_data) numero_label.grid(row=1, column=0, padx=5, pady=5) numero_entry.grid(row=2, column=0, padx=5, pady=5)
3️⃣ The third part is creating the Comboboxes. These elements are like drop-down lists seen in web development or others.
To create them in Python you must give it a name followed by ttk.Combobox().
Then, you have to block the user from writing above the list with ttk.Combobox(state=”readonly”)
Finally, you must write all the values that you want to appear in said Combobox. In this case, it will be all the currencies available in the API.
This must be repeated twice, first for the currency of your money and then the currency you want to convert it to.
combo = ttk.Combobox() combo = ttk.Combobox(state="readonly") combo["values"] = ["USD", 'AED', 'ARS', 'AUD', 'BGN', 'BRL', 'BSD', 'CAD', 'CHF', 'CLP', 'CNY', 'COP', 'CZK', 'DKK', 'DOP', 'EGP', 'EUR', 'FJD', 'GBP', 'GTQ', 'HKD', 'HRK', 'HUF', 'IDR', 'ILS', 'INR', 'ISK', 'JPY', 'KRW', 'KZT', 'MVR', 'MXN', 'MYR', 'NOK', 'NZD', 'PAB', 'PEN', 'PHP', 'PKR', 'PLN', 'PYG', 'RON', 'RUB', 'SAR', 'SEK', 'SGD', 'THB' 'TRY', 'TWD', 'UAH', 'UYU', 'ZAR'] combo.grid(row=3, column=0, padx=5, pady=5) second_moneda = Label(ventana, text="A qué moneda lo quieres convertir?") second_moneda.grid(row=4, column=0, padx=5, pady=5) combo2 = ttk.Combobox() combo2 = ttk.Combobox(state="readonly") combo2["values"] = ["USD", 'AED', 'ARS', 'AUD', 'BGN', 'BRL', 'BSD', 'CAD', 'CHF', 'CLP', 'CNY', 'COP', 'CZK', 'DKK', 'DOP', 'EGP', 'EUR', 'FJD', 'GBP', 'GTQ', 'HKD', 'HRK', 'HUF', 'IDR', 'ILS', 'INR', 'ISK', 'JPY', 'KRW', 'KZT', 'MVR', 'MXN', 'MYR', 'NOK', 'NZD', 'PAB', 'PEN', 'PHP', 'PKR', 'PLN', 'PYG', 'RON', 'RUB', 'SAR', 'SEK', 'SGD', 'THB' 'TRY', 'TWD', 'UAH', 'UYU', 'ZAR'] combo2.grid(row=5, column=0, padx=5, pady=5)
4️⃣ We continue with the fourth step, which is to make the button with the command of a function that we will do in the next step. Function that will perform all the conversion logic and display the result.
Also, let's not forget ❗ our precious mainloop. For the program to appear visually.
boton = Button(ventana, text="Convertir", command=conversion) boton.grid(row=6, column=0) ventana.mainloop()
5️⃣ In our fifth step we are going to create a function with the name that we have given to the property command of our buttton. def conversion():
Within this function, we are going to do a try/except mainly for when the user inserts something other than numbers.
TRY
We are going to create a variable to obtain the URL of the API adding at the end the currency number 1 with combo.get().
Next, we create a response variable so that it obtains the requests from the url with requests.get(url).
Next, we create another data variable to store the response json.
And now, we start with the math! 🔣
We have to pass the number we have to float, creating a new variable.
To finish, we must create a new result variable by multiplying said float number by the existing currency in the JSON file.
That said, we would only need to establish a design and voila.
EXCEPT
We are simply going to copy the design made for the previous result, putting text over the typo and making it more striking.
def conversion(): try: url = 'https://v6.exchangerate-api.com/v6/TU-API-KEY/latest/'+combo.get() response = requests.get(url) data = response.json() numero1 = float(numero_entry.get()) resultado = round((numero1 * data['conversion_rates'][combo2.get()]), 2) espacio = Label(ventana, text="") espacio.grid(row=7, column=0) resultado_label = Label(ventana, text=resultado) resultado_label.grid(row=8, column=0) resultado_label.config( fg="black", bg="white", font=("Arial", 20), padx=400, pady=20 ) except: espacio = Label(ventana, text="") espacio.grid(row=7, column=0) resultado_label = Label(ventana, text="Hay algo incorrecto. Recuerda que solo puedes escribir NÚMEROS") resultado_label.grid(row=8, column=0) resultado_label.config( fg="red", bg="black", font=("Arial", 20), padx=100, pady=20 )
If we haven't skipped any steps, our project should look something like this:
And that's all for today guys, I hope you liked this post as much as I liked doing it, programming the project and fighting with the API! After all, this is the beauty of programming, don't you think? 🤓
See you here very soon and you already know that you can follow me on Twitter, Facebook, Instagram and LinkedIn. 🤟🏻