Hello world 🤟🏻 my name is Francisco, fcoterroba on the Internet and today I bring you a post that you usually like here on the web. As you see in the title, today we are going to create an API in Python using the FastAPI library or framework.
Very interesting project to add to your developer portfolio, whether backend or even front-end.
Before starting, how could it be otherwise, if you haven't seen it yet, I strongly recommend that you go watch the latest video I have on my YouTube channel in which I explained, in a very simple and concise way, how to perform the installation of a smart light bulb 🧠 with which you can change its color, control it with your own voice and much more! You can see it here 👇🏻
Finally, before starting, I wanted to communicate that I have been uploading videos to TikTok for a few weeks. Lately they are from Eurovision since there is little left for it but I leave you here the last one I did today on computers.
@fcoterroba ¿Cómo hacer un pequeño #juego en #Python ? Like para parte 2! #fyp #parati #dev #programacion ♬ LoFi(860862) – skollbeats
Tabla de contenidos
¿Anything I should know before I start?
Mmm, yep, some things really:
- What is an API?
- An API (short for Application Programming Interfaces), is, basically, a set of definitions and protocols that are used to develop and integrate application software or websites. Thus allowing communication between two a priori totally different applications.
- An API is a place where certain information about certain aspects is contained. The thing is that such information is not easily accessible and readable by humans.
- Generally, an API returns the information when it is required in JSON format and it is the developer of their new website or software who is in charge of making said information readable.
- For example, this free API from The Simpsons, if you access the basic API, it returns a phrase from one of the characters in this series.
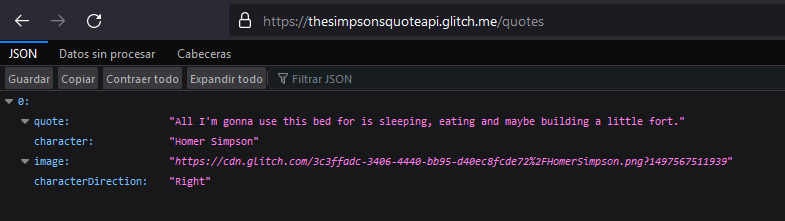
- What is Python?
- Python is a programming language used in practically everything imaginable.
- Web development, software, AI, ML, Data Science. These are some of the tech areas in which Python is widely used.
- It offers quite good readability and is close to human reading.
- In the category Python On the web you can find +8 projects with Python divided by their difficulty. What are you waiting for? Your API can wait.
- What is FastAPI?
- FastAPI is a framework to build APIs easily and quickly with Python.
- In recent times it has become a very popular framework as it is considered one of the fastest existing frameworks in Python.
- Some benchmarks have even include FastAPI a lot more forwarding Django, Flask or Pyramid.

1️⃣ FIRST STEP 1️⃣ Install what we need
We are going to keep in mind that we already have Python installed, in my case I have version Python 3.10.7 but you do not necessarily have to have this version to follow the tutorial. It is only recommended that it be a Python 3 version. python 3.x.x
The first thing we have to do is install the FastAPI library, for this we are going to use the command
pip install fastapi
Lastly, we will need the library uvicorn, an ASGI web server written for Python. We will need this library to check that everything is working. We can install it with this command:
pip install uvicorn
Once installed, we can really start
2️⃣ SECOND STEP 2️⃣ Hello World
The first thing, and as you can already imagine, is to import the FastAPI library, for this we import it like any other:
from fastapi import FastAPI
Then, we will have to create a general object that is usually called an app
app = FastAPI()
FastAPI works with asynchronous functions and many getters, so we will have to take, to begin with, the root of our server and declare the return message below:
from fastapi import FastAPI app = FastAPI() @app.get("/") async def root(): return {"message": "Hello World"}
With this, if we view our localhost (by default on port 8000), we will obtain the json that we have sent to return:
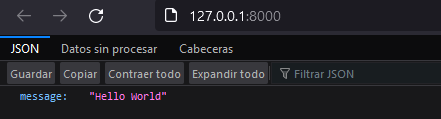
3️⃣ THIRD STEP 3️⃣ More functions and pages
With the previous step we have made great progress, we now have our web server working, waiting for the query and it returns us a json with what we say. It's okay, but we need more things, right?
In this case I am going to do the test as if I were creating an API of the famous anime “Shin Chan”.
The first thing we will have to do is, in some way, store the information that we will want to return in the API. I am going to store the main characters of the series in a JSON with their age, an image, name, etc. Like this:
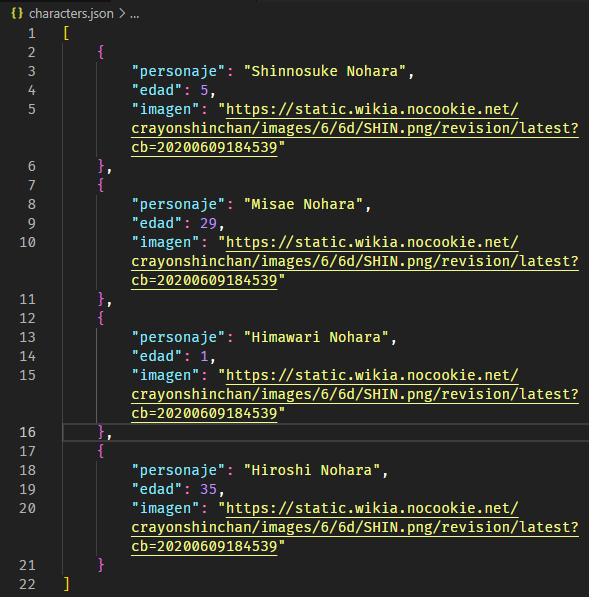
Now, let's say we want to pass those four characters to the root of our API.
To do this we will simply have to convert our JSON file to a Python dictionary. We import the json library to work with it and opening it, we can pass the variable using the load function
import json data = "" with open('characters.json') as json_file: data = json.load(json_file)
Finally, in our root we pass this variable and if we visit our API we will obtain said json
@app.get("/") async def root(): return data
If we pass, for example, data[0] we'll just give the first value of json data[1] for the second one, etc.
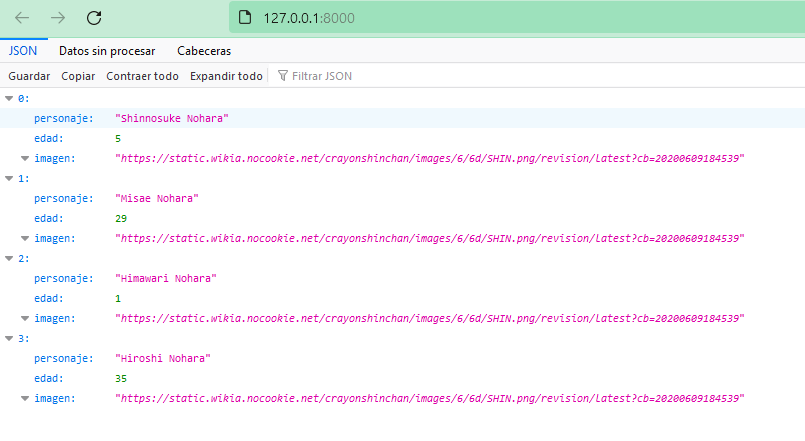
Now, all this we have done has been in root, when accessing the main page of the API, suppose we want a certain thing to be returned on each page.
To do this, we will have to create a getter with a function for each page we want.
For example, let's say I want a page that will return a completely random character for each visit. We import the random library and use the choice function passing our dictionary AS LIST.
import random @app.get("/randomCharacter") async def randomCharacter(): return random.choice(list(data))
Another example, suppose we have a list with all the ids that we can obtain, for this we can pass an id to the getter and the function, to return said record.
Note that we subtract one because the json starts with 0 but the user will write “normal”, starting with 1.
@app.get("/specificCharacter/{id}") async def specificCharacter(id: int): return data[id-1]
4️⃣ FOURTH STEP 4️⃣ Main page 4️ -
We are almost finished, we already have our basic API, our functions, our calls, everything.
But of course, our root continues to return a json, it is neither aesthetic nor simple nor does it have good practices. The correct thing to do will be to create an index.html and call said file in root.
I have created this small 50-line HTML based on a W3Schools template.
<!DOCTYPE html> <html> <head> <title>The Shin Chan API - @fcoterroba</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://www.w3schools.com/w3css/4/w3.css"> <link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Raleway"> <style> body,h1,h2{font-family: "Raleway", sans-serif} body, html {height: 100%} p {line-height: 2} .bgimg { min-height: 100%; background-position: center; background-size: cover; } .bgimg {background-image: url("https://preview.redd.it/7fx1jell8p551.png?auto=webp&s=8ff53edc7ff4752ca0262c8a58f2d0a7efbbad61")} </style> </head> <body> <header class="w3-display-container w3-wide bgimg w3-grayscale-min" id="home"> <div class="w3-display-middle w3-text-blue w3-center" style="background-color: white;"> <h1 class="w3-jumbo">Shin Chan API</h1> <h2>The free Shin Chan API</h2> <h2><b>Created by @fcoterroba</b></h2> </div> </header> <div class="w3-container w3-padding-64 w3-pale-blue w3-grayscale-min" id="us"> <div class="w3-content"> <h1 class="w3-center w3-text-grey"><b>Just call it</b></h1> <img class="w3-round" src="https://images5.alphacoders.com/116/1166283.jpg" style="width:100%;margin:32px 0"> <p><i>Get the full name, age and picture of your favourite characters.</i> </p><br> <p class="w3-center"><a href="#" class="w3-button w3-black w3-round w3-padding-large w3-large">Documentation</a></p> </div> </div> </body> </html>
Once we have the html, we simply import the function FileResponse from starlette.responses and we return it using said function.
from starlette.responses import FileResponse @app.get("/") async def root(): return FileResponse('index.html')
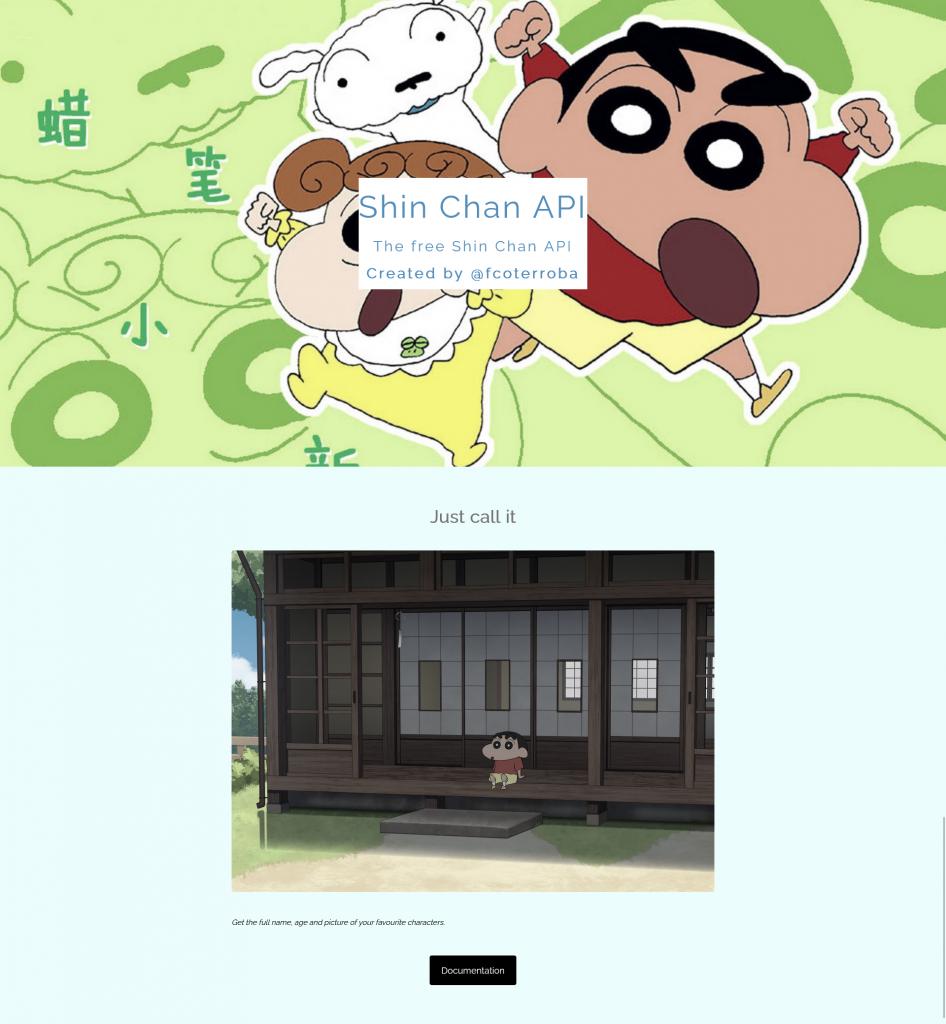
5️⃣ FIVETH STEP 5️⃣ Generate documentation
Any API worth its salt requires custom documentation and in that FastAPI also has a very good point in its favor.
By default, this library generates two very clean and beautiful documentations
One of them is in /redoc and the other, somewhat more classic, in /docs.
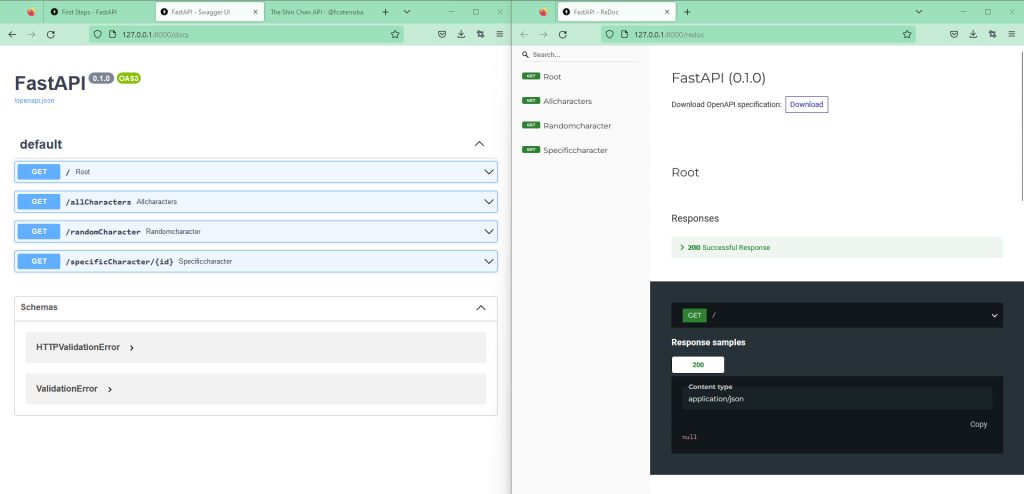
You can see my API's repo in GitHub!
🏁 END OF THE POST 🏁
And this has been all for today. Thank you for reaching the end, I hope you have served and liked it. See you soon!
You can contribute financially through Paypal. Any amount is well received! 🙂
I also hope you have a great week and see you here soon! A greeting and remember to follow me on the networks as Twitter, Facebook, Instagram, GitHub, LinkedIn and now too in TikTok. 🤟🏻
sources: Xataka.com, AWS.amazon.com, sngular.com, coffeebytes.dev, w3schools.com